Tokenization using i-Frames
How Jupico utilizes a Token Element to securely capture cardholder data and create an always up-to-date token for future use.
Understanding PCI DSS
To understand why tokenization plays a critical role in the payments industry, you first need to understand PCI Compliance:
PCI DSS (Payment Card Industry Data Security Standards) is mandated by credit card companies to help ensure the security of credit card transactions in the payments industry. PCI compliance refers to the technical and operational standards that businesses follow to secure and protect credit card data provided by cardholders and transmitted through card processing transactions.
Every integrator must define what level of PCI compliance will be required through a self assessment questionnaire (SAQ).
To reduce time, effort, and the scope of PCI, Jupico provides developers with a safe standard to capture and store credit and debit card-sensitive data through tokenization.
Jupico's Tokenization Method
Tokenization basics
Tokenization is the process of replacing sensitive data with unique identification symbols that retain all the essential information about the data without compromising its security.
Jupico uses tokenization to capture sensitive credit and debit card data, including personally identifiable information (PII), through a collection of specialized web and mobile UI components that are responsible for sending the raw data to a secure vault and returning a unique token.
How it works
Jupico intercepts sensitive data before it reaches your server and replaces it with aliased versions, securing the original information in our vault.
All data in motion from the web browser (VGS-Collect i-frames) to VGS-Vault is sent over TLS 1.2 with Authenticated Encryption mode ciphers. The data at rest (in the Vault) is tokenized and encrypted at rest via AES-256-GCM.
- This process typically occurs while creating the user profile or during the checkout flow.
- Tokenization allows your platform or marketplace to operate in a PCI compliant environment while remaining out of PCI scope.
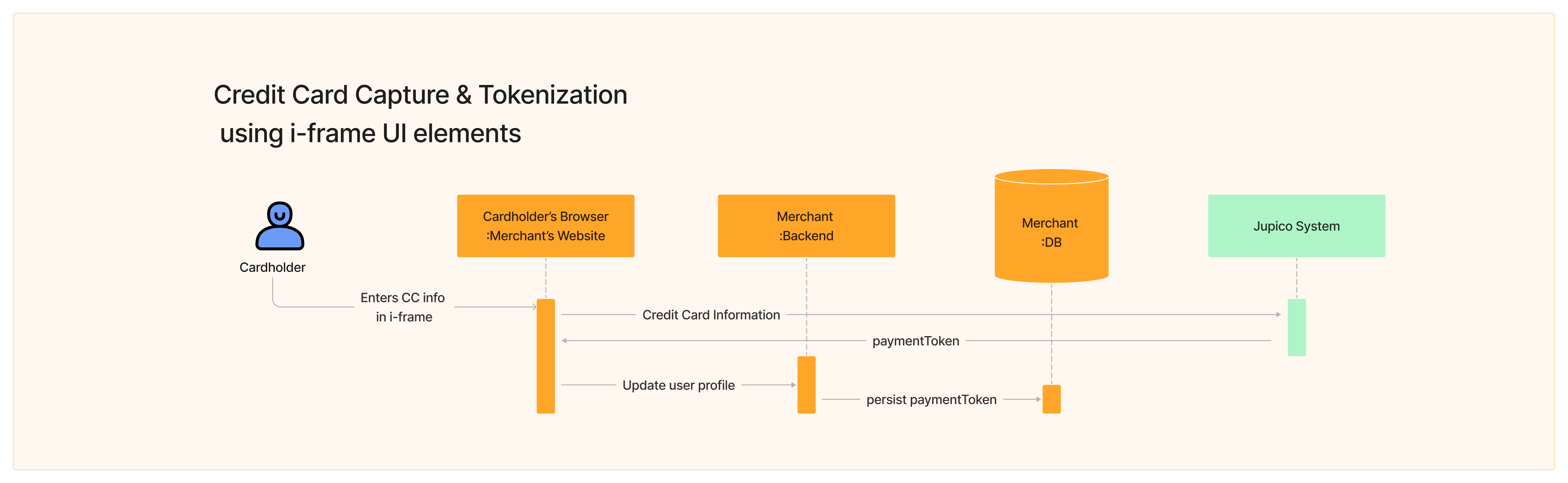
- Integrate Jupico's JavaScript Library
- Embed Jupico's JavaScript (found below) into your webpage to dynamically generate a secure form for capturing credit card details.
- Secure Data Transmission
- Upon form submission, the sensitive credit card information is securely sent to Jupico's encrypted vault.
- Token Retrieval
- A JSON token is returned to your application, which you can store for future use or transaction processing.
Credit Card Tokenization with UI components
Include Jupico's JavaScript (VGS collect UI components) in your application to safely capture credit card information. This script will validate and send the sensitive data directly to Jupico Platform, ensuring it never touches your servers and freeing you up from PCI compliance.
// VGS Collect form initialization
const tokenForm = VGSCollect.create('tntne5koztu', function(state) {
// Function to check the tokenForm state
});
// Create VGS Collect field for credit card name
tokenForm.field('#cc-name', {
type: 'text',
name: 'CardHolderName',
validations: ['required'],
});
// Create VGS Collect field for credit card number
tokenForm.field('#cc-number', {
type: 'card-number',
name: 'CreditCardNumber',
successColor: '#4F8A10',
errorColor: '#D8000C',
validations: ['required', 'validCardNumber'],
});
// Create VGS Collect field for CVC
tokenForm.field('#cc-cvc', {
type: 'card-security-code',
name: 'Cvv',
validations: ['required', 'validCardSecurityCode'],
});
// Create VGS Collect field for credit card expiration date
tokenForm.field('#cc-expiration-date', {
type: 'card-expiration-date',
name: 'ExpirationDate',
serializers: [tokenForm.SERIALIZERS.separate({monthName: 'ExpirationMonth', yearName: 'ExpirationYear'})],
validations: ['required', 'validCardExpirationDate']
});
// submit tokenForm
let resp = await fetch("https://dev-platform.jupiterhq.com/v1/transactions/creditcard/tokenization/874767775/session");
let sessionToken = await resp.body.text();
let sessionData = await resp.json();
let sessionId;
if (sessionData.data && sessionData.data.status === "active") {
sessionId = sessionData.data.sessionId;
}
//submit cc fields to VGS for tokenization
form.submit(
"/v1/transactions/creditcard/tokenization/" + subMerchantId,
{ data: { sessionToken: sessionId }},
tokenizationComplete(status, data))
// Tokenization complete callback
function tokenizationComplete(status, tokenizationData) {
//check response status
if(status === 200) {
//perform any operation that you need
alert(JSON.stringify(tokenizationData));
}
}
<div id="cc-container-wrap">
<div class="form-group">
<label for="cc-name">Name</label>
<span id="cc-name" class="form-field">
<!--VGS Collect iframe for card name field will be here!-->
</span>
</div>
<div class="form-group">
<label for="cc-number">Card number</label>
<span id="cc-number" class="form-field">
<!--VGS Collect iframe for card number field will be here!-->
</span>
</div>
<div class="form-group">
<label for="cc-cvc">CVC</label>
<span id="cc-cvc" class="form-field">
<!--VGS Collect iframe for CVC field will be here!-->
</span>
</div>
<div class="form-group">
<label for="cc-expiration-date">Expiration date</label>
<span id="cc-expiration-date" class="form-field">
<!--VGS Collect iframe for expiration date field will be here!-->
</span>
</div>
</div>
#cc-container-wrap body {
padding: 25px;
}
#cc-container-wrap span[id*="cc-"] {
display: block;
height: 40px;
margin-bottom: 15px;
}
#cc-container-wrap span[id*="cc-"] iframe {
height: 100%;
width: 100%;
}
#cc-container-wrap pre {
font-size: 12px;
}
#cc-container-wrap .form-field {
display: block;
width: 100%;
height: calc(2.25rem + 2px);
padding: 0.375rem 0.75rem;
font-size: 1rem;
line-height: 1.5;
color: #495057;
background-color: #fff;
background-clip: padding-box;
border: 1px solid #ced4da;
border-radius: 0.25rem;
transition: border-color 0.15s ease-in-out, box-shadow 0.15s ease-in-out;
}
#cc-container-wrap .form-field iframe {
border: 0 none transparent;
height: 100%;
vertical-align: middle;
width: 100%;
}
#cc-container-wrap p {
margin-bottom: 10px;
}
Updated about 1 month ago